Make your Ruby on Rails app work faster using Rails caching. When you use Rails cache, your app responds quicker, needs less from the database, and makes users happier. Let's learn more about this in our tutorial guide.
Introduction
Did you know that if a page takes one second longer to load, customers might be 16% less happy?
Do you want your app to respond super fast to users?
That's where Rails caching comes in. It's the best way to make your app faster and give users a smooth experience. This technique saves or uses data already made to make things faster.
You can make your app work better by using a cache. Let's see why it's good to use Rails caching, learn about the types of caches, how to use different stores, clear it, and see some real examples.
Why Use Rails Caching?
Rails caching helps apps work better and handle more users. Here's why it's so useful:
Boost Responding Process
Rails caching makes apps faster by saving and showing pre-made content. It avoids repeating things or doing hard math. It directly gives users what they need, making pages load faster and giving a smooth app experience.
Increase Application Capabilities
When you use Rails caching, your Ruby on Rails app works better and deals well with lots of tasks. It gets important info faster from a cache, so it can give users what they need quicker, making the app easier to use.
Cost Optimization
Using a Rails cache can save money for your RoR app. By using resources better, you get rid of unneeded databases and focus on what's important. It means fewer servers and hosting that can make the app slow. Using fewer resources can cut down on how much it costs to run the app.
Less Dependency On Third-Party API
Cache helps by keeping data or responses from external APIs close by. When you have the API response stored, you ask for fewer things from outside services. This means fewer chances of things going wrong.
Enhance Backend Performance
Rails caching is great because it helps the backend work smoothly. It does this by handling data and storing hard operations. This makes the app easier to use, faster to load, and able to grow better.
Different Types of Rails Cache
Here are different ways Rails caching makes apps work faster and better.
Page Caching
Page caching helps pages load faster by letting saved pages be shown without doing all the work again in Rails. Servers like Apache and NGINX help with this.
But it can't be used in all places, like pages needing logins or specific files. Also, if a page needs a login or has secret info, we can't use page caching for it.
We can use page caching like this:
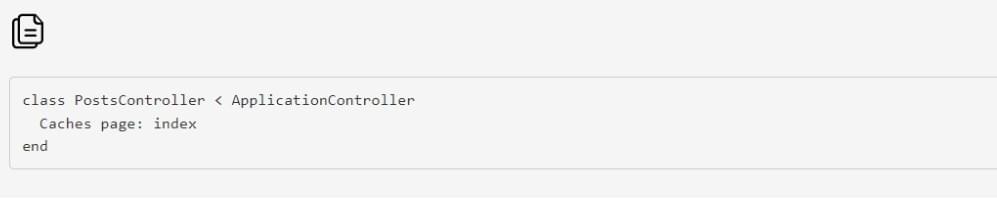
Making sure cached pages are up-to-date is important for managing page caching in Ruby on Rails. Even though caching makes things faster, it's essential to show the newest information.
Cache expiration decides how long a cached page stays correct before it needs to be updated. The time it stays valid depends on how often the content changes.
In Rails, we can decide how long cached pages stay valid. We can set one time for all pages in the 'config/environments/production.rb' file or set different times for specific actions using the expires_in method.

Action Caching
Action caching is like page caching but solves some issues. In page caching, things like authentication don't work because it sends data from the web server.
But in action caching, things like authentication can work before showing the data. With action caching, the request goes through the Rails stack, allowing checks like validation and authentication to happen when needed.
Rails action caching helps by saving the results of actions, such as 'index' and 'show', in a cache.
Here is an example of it:
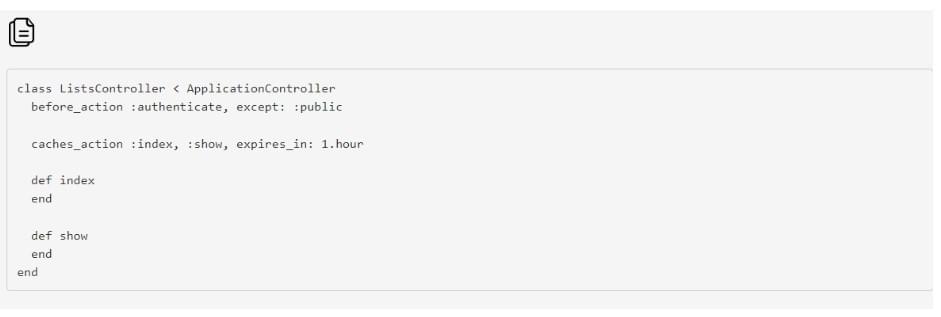
Low-level Caching
In Rails, we can do low-level caching by using Rails.cache.fetch.
The code:
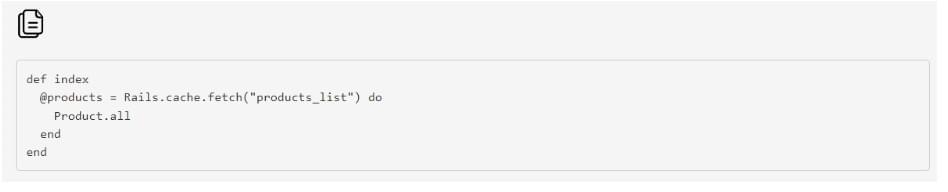
Here’s how Rails.cache.fetch works:
First, find the specific cache data and create a key for it (like 'products_list'). Then, check if this data is already stored in the cache.
If the data exists in the cache, Rails.cache.fetch gives back the stored data immediately.
If the data isn't in the cache, Rails.cache.fetch runs the code block that you gave as a second step.
Afterward, the outcome of the block of code is saved in the cache using the specific cache key.
Rails.cache.fetch also gives back the result, so you can use the obtained data in your app.
Low-level caching helps us save particular parts of code or data, giving us better control over how we cache things. This is handy in Rails for caching small but often used data, like database searches or complicated calculations.
Advanced Rails Caching Techniques
To make your app faster and better, you can try these special ways of caching in Rails:
Fragment Caching
Fragment caching saves a specific part of a page. Let's say you have a blog with a sidebar showing recent posts. Instead of getting this list every time from the database, you can save it once and reuse it when needed. In simple terms, it's like saving a piece of the webpage so you can use it later without fetching it again and again.
Russian Doll Caching
In Ruby on Rails, Russian Doll caching helps us save different parts of a webpage in layers, like nesting dolls. Let's say you have a blog with posts and comments. Each post has several comments. Instead of fetching and showing all comments every time, this caching lets you save them in layers, like a set of nesting dolls. So, when you need them, you can quickly get the whole set without doing the work every time.
Examples of when to use Rails cache
These are actual instances of applying Rails cache in your apps. These are stories about how Rails caching helped and succeeded.
GitHub
GitHub, the code-sharing site, used page caching to save public repository pages. This helped GitHub give fast and smooth experiences to many users worldwide.
Airbnb
Airbnb, a vacation rental app, used fragment caching to get different parts of its app. This helped Airbnb show improved search results, property lists, and made it easier to create user profiles.
SoundCloud
SoundCloud, an online music service, uses fragment caching to improve different parts of its platform. This helps SoundCloud show better track lists, make playlists faster, and have a large search collection while making the site quicker to respond.
Shopify
A shopping website, Shopify, uses different types of caching (page, low-level, and fragment) to make its site work better. By using caches, Shopify makes the search, shopping cart, and product details load faster for customers.
Conclusion
Don't settle for average app speed when Rails caching can make your Ruby on Rails app work much better. With the right caching methods, your app will load faster, have less downtime, solve the N+1 query issue, and reduce the database workload.
Consider hiring a Ruby on Rails developer or a Ruby on Rails development company to create solutions that suit your needs and work efficiently.